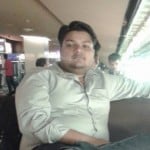
Gourav Verma
IndividualForum Replies Created
-
Gourav
MemberAugust 26, 2016 at 8:36 am in reply to: What is the difference between the multiple messaging options in Visualforce?Hi Tanu,
Let me start off by explaining each of these in more detail.
apex:pageMessage is used to display a single custom message using the Salesforce formatting. You can specify the severity (which will control the display of the message box) and the strength (which will control the size of the box). Take the following code for example:
<apex:page >
<apex:pageMessage summary="This is a pageMessage" severity="info" strength="3"/>
</apex:page>Now let's tweak that code slightly:
<apex:page >
<apex:pageMessage summary="This is a pageMessage" severity="error" strength="1"/>
</apex:page>Notice how I changed the severity and the strength. This controls the size and the display of the message.
This can be used to display any custom message that you always want to appear on the screen. There may be a case that whenever a user is on a form you may want a warning to appear to display important information to them.
apex:pageMessages is used to display all of the messages on a page. It will display Salesforce generated messages as well as custom messages added to the ApexPages class. Let's take a look at it in use:<apex:page controller="TestMessageController">
<apex:pageMessages />
</apex:page>public class TestMessageController{
public TestMessageController(){
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.ERROR, 'This is apex:pageMessages'));
}
}Let's add some more messages and see how it reacts:
<apex:page controller="TestMessageController">
<apex:pageMessages />
</apex:page>public class TestMessageController{
public TestMessageController(){
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.ERROR, 'This is apex:pageMessages'));
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.ERROR, 'This is apex:pageMessages still'));
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.INFO, 'This is apex:pageMessages info'));
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.INFO, 'This is apex:pageMessages warning'));
}
}Notice how that single instance of the apex:pageMessages handles all of the errors on the page. The use case for this object is to capture all errors on the page. It should be used on most custom Visualforce page as a catch all to let users know what errors occur (if no other messaging is used).
apex:message is used to display an error on only a very specific field. It is used to allow the developer to place field specific errors in a specific location. Take the following code for example:<apex:page controller="TestMessageController">
<apex:form >
<apex:outputLabel value="Test String" /><apex:inputText value="{!test.Name}" id="test"/>
<br/><apex:message for="test"/>
</apex:form>public class TestMessageController{
public Account test{get;set;}
public TestMessageController(){}
}Notice how this currently doesn't have a message displayed. Also notice how this particular element is specified specifically for one field. The error will only occur on that one field. Let's change the code a bit and take a look at this with an error on the Name field:
<apex:page controller="TestMessageController">
<apex:form >
<apex:outputLabel value="Test String" /><apex:inputField value="{!test.Name}" id="test"/>
<br/><apex:message for="test"/>
</apex:form>
</apex:page>public class TestMessageController{
public Account test{get;set;}
public TestMessageController(){
test = new Account();
test.Id.addError('Correct');
test.Name.addError('Wrong');
}
}Notice how only the Name error is shown because that is the only field that has an apex:message associated to it. Also notice that although this does have some styling, the styling is minimalistic and Salesforce expects the developer to handle the styling as necessary in this situation.
The final thing to look at is apex:messages. apex:messages is similar to apex:message, but it displays all of the errors. These errors are displayed as a list with no styling. Let's take the last example we used in the apex:message and simply add the apex:messages tag to the Visualforce. The code:<apex:page controller="TestMessageController">
<apex:messages />
<apex:form >
<apex:outputLabel value="Test String" /><apex:inputField value="{!test.Name}" id="test"/>
<br/><apex:message for="test"/>
</apex:form>
</apex:page>public class TestMessageController{
public Account test{get;set;}
public TestMessageController(){
test = new Account();
test.Id.addError('Correct');
test.Name.addError('Wrong');
}
}This provides no formatting for the errors, however, it does display them all. The use case for using apex:messages is to display all errors on the page while providing your own styling.
Now, to close it all out, let's take a look at them all together. The code:<apex:page controller="TestMessageController">
<apex:pageMessages />
<apex:pageMessage summary="This is apex:message" severity="info" strength="2"/>
<apex:messages />
<apex:form >
<apex:outputLabel value="Test String" /><apex:inputField value="{!test.Name}" id="test"/>
<br/><apex:message for="test"/>
</apex:form>
</apex:page>public class TestMessageController{
public Account test{get;set;}
public TestMessageController(){
ApexPages.addMessage(new ApexPages.Message(ApexPages.Severity.ERROR, 'This is apex:pageMessages'));
test = new Account();
test.Id.addError('Correct');
test.Name.addError('Wrong');
}
}As expected, apex:pageMessages shows all of the errors with formatting, apex:pageMessage only shows the specified message, apex:messages shows all of the errors with no formatting, and apex:message shows only the error for the specified field.
Note :- Copy past these codes in VF page and saw the diffrence.
-
Gourav
MemberAugust 26, 2016 at 8:28 am in reply to: How can we avoid the trigger from firing by a process builder?From what it seems you are dealing with a recursion problem here. The most obvious solution I can think of is using some custom settings or using a helper class that would enable the execution of the trigger one time and that would become inactive after the execution.
This would guarantee that your trigger is only being executed once.
This is an example of a helper class
public Class checkRecursive{
private static boolean run = true;
public static boolean runOnce(){
if(run){
run=false;
return true;
}else{
return run;
}
}
}And this is in action
trigger updateTrigger on anyObject(after update) {
if(checkRecursive.runOnce())
{
//write your code here
}}
The above example was taken from here : link
-
Hi Tanu,
Yes, you can accessed reports in apex.
Firstly, you can get a list of reports in XML from: https://instance.salesforce.com/servlet/servlet.ReportList (replacing instance with the actual server instance, such as na1)
Once you have extracted the required report Id (00O key prefix) you can then request the report data in either CSV or HTML.
CSV https://instance.salesforce.com/00Ox0000000xxxx?export=1&enc=UTF-8&xf=csv
HTML https://instance.salesforce.com/00Ox0000000xxxx?export=1&enc=UTF-8&xf=xls (Last time I checked this was HTML rather than a native Excel file format. Excel does the conversion when it is opened).As for working with the CSV output, I found Parse a CSV with APEX but I haven't tested it yet.
If you want to get fancy, you can set filters for the report too. See BUILDING SALESFORCE CUSTOM LINKS - Linking to Reports for the pv0, pv1... query string parameters.
Reference:
Progmatic Access to Salesforce.com Reports This post was from 2006, but shows that the approach has been working for a several years now. That still doesn't mean it might not work tomorrow.
-
Gourav
MemberJuly 5, 2016 at 2:23 pm in reply to: Disable commandButton after first click to prevent double submissionHi Himanshu,
You can try this approach
<apex:pageBlockButtons>
<apex:actionStatus id="status" >
<apex:facet name="start">
<apex:outputPanel >
Merging... <apex:image value="{!URLFOR($Resource.blueSquares)}"/>
</apex:outputPanel>
</apex:facet>
<apex:facet name="stop">
<apex:outputPanel >
<apex:commandButton value="Merge" action="{!mergeOpps}" rerender="error1,error2" status="status"/>
<apex:commandButton value="Cancel" action="{!cancel}" immediate="true"/>
</apex:outputPanel>
</apex:facet>
</apex:actionStatus>
</apex:pageBlockButtons>In this we use apex:actionStatus for this.
Hope this will help.
-
Completed date show when the job is done or completed if job is in progress or not completed or shedule for future then it can't display. So check if your apex job is done or not.
-
Gourav
MemberJune 20, 2016 at 3:24 pm in reply to: Do we need to integrate any 3rd party to implement Open CTI in Salesforce?Salesforce CRM Call Center seamlessly integrates Salesforce with third-party computer-telephony integration (CTI) systems. Before the introduction of Open CTI, Salesforce users could only use the features of a CTI system after they installed a CTI adapter program on their machines. Yet such programs often included desktop software that required maintenance and didn’t offer the benefits of cloud architecture. Open CTI lets developers:
- Build CTI systems that integrate with Salesforce without the use of CTI adapters.
- Create customizable SoftPhones (call-control tools) that function as fully integrated parts of Salesforce and the Salesforce console.
- Provide users with CTI systems that are browser and platform agnostic, for example, CTI for Microsoft® Internet Explorer®, Mozilla® Firefox®, Apple® Safari®, or Google Chrome™ on Mac, Linux, or Windows machines.
Developers use Open CTI in JavaScript to embed API calls and processes; Open CTI is only available for use with JavaScript pages. To use Open CTI, developers should have a basic familiarity with:
- CTI
- JavaScript
- Visualforce
- Web services
- Software development
- The Salesforce console
- Salesforce CRM Call Center
-
Gourav
MemberJune 20, 2016 at 3:22 pm in reply to: What are the best practices to implement the email deliverability settings in salesforce?To improve the deliverability of email you send from Salesforce, configure your organization's email deliverability settings. Email deliverability is the likelihood of a company’s or individual’s email reaching its intended recipient. This likelihood is adversely affected by:
Bounced email
Email that is addressed to an invalid recipient and returned to the sender. If a sender sends several email messages that bounce, the email server might slow or block the delivery of all email from that sender.
Noncompliant email
Email that does not comply with a recipient's email security framework, such as the Sender Policy Framework (SPF), which verifies that the From address in an email message is not forged.To configure the email deliverability settings for your organization:
- From Setup, enter Deliverability in the Quick Find box, then select Deliverability.
- To control the type of email your organization sends, change the Access level in the Access to Send Email section. You may not be able to edit the Access level if Salesforce has restricted your organization’s ability to change this setting.
- No access: Prevents all outbound email to and from users.
- System email only: Allows only automatically generated emails, such as new user and password reset emails.
- All email: Allows all types of outbound email. Default for new, non-sandbox organizations.
- Select the Activate bounce management checkbox to help ensure that the email addresses you have for your contacts, leads, and person accounts are correct, and that the email your users send to those addresses is not impeded due to excessive email bounces.When bounce management is activated and a user sends an email to a contact, lead, or person account with an invalid email address, Salesforce displays an alert next to that email address and prevents users from sending email to the address until it is updated or confirmed. Also, the email bounces to Salesforce instead of the sender's personal email account.
- Select the Show bounce alert next to all instances of the email address checkbox to configure Salesforce to search all lead, contact, and person account records for instances of any email address that bounces an email and to display a bounce alert next to each instance. If you do not select this option, Salesforce only displays the bounce alert on the record from which the email was originally sent.
- Select the Return bounced email to sender checkbox to configure Salesforce to send a copy of the bounced email header to the sender. If you do not select this option, only Salesforce receives the bounced email header. In either case, for security purposes Salesforce does not return the body of the bounced email to the sender. This option applies to all users in your organization and cannot be enabled per user or per email.
- Select the Enable compliance with standard email security mechanisms checkbox to automatically modify the envelope From address of every email you send from Salesforce to comply with email security frameworks that your recipients might implement, such as SPF.Many recipient email systems enforce SPF to verify whether an email is legitimate. SPF checks the envelope From address of an inbound email to verify legitimacy. If this feature is enabled, Salesforce changes the envelope From address to a Salesforce email address to verify the sender's legitimacy. The header From address remains set to the sender's email address.
- Select the Enable Sender ID compliance checkbox to comply with the Sender ID framework. This will automatically populate the Sender field in the envelope of every email you send from Salesforce with no-reply@Salesforce. This enables receiving mail servers using the Sender ID email authentication protocol to verify the sender of an email by examining the Senderand From headers of an inbound email through a DNS lookup. All replies will still be delivered to the sender's email address. If you do not select this checkbox, the Sender field is set to null and email delivery fails when a recipient email system performs a Sender ID check.
- If you want Salesforce to send users a status email when their mass emails are complete, select Notify sender when mass email completes.
- To specify how Salesforce uses the Transport Layer Security (TLS) protocol for secure email communication for SMTP sessions, choose one of the following:
- Preferred (default): If the remote server offers TLS, Salesforce upgrades the current SMTP session to use TLS. If TLS is unavailable, Salesforce continues the session without TLS.
- Required: Salesforce continues the session only if the remote server offers TLS. If TLS is unavailable, Salesforce terminates the session without delivering the email.
- Preferred Verify: If the remote server offers TLS, Salesforce upgrades the current SMTP session to use TLS. Before the session initiates, Salesforce verifies the certificate is signed by a valid certificate authority, and that the common name presented in the certificate matches the domain or mail exchange of the current connection. If TLS is available but the certificate is not signed or the common name does not match, Salesforce disconnects the session and does not deliver the email. If TLS is unavailable, Salesforce continues the session without TLS.
- Required Verify: Salesforce continues the session only if the remote server offers TLS, the certificate is signed by a valid certificate authority, and the common name presented in the certificate matches the domain or mail exchange to which Salesforce is connected. If any of these criteria are not met, Salesforce terminates the session without delivering the email.3
- Click Save.
-
Gourav
MemberJune 20, 2016 at 2:53 pm in reply to: How to create a mobile app using mobile SDK in Salesforce?The Salesforce Mobile SDK for Android is an open source toolkit that provides a collection of native libraries to enable developers to rapidly build Android applications that securely connect to the Salesforce Platform. The SDK abstracts the underlying OAuth 2.0 and REST API calls needed to connect an Android application with the Salesforce Platform, allowing developers to focus on their app's functionality.
The SDK also provides a hybrid container based on the open source Apache Cordova (PhoneGap) project that enables HTML5-based applications to leverage device features like the camera and microphone. The hybrid SDK extends the core Cordova platform to provide additional libraries for key enterprise requirements such as OAuth 2.0 authentication and secure offline storage, effectively providing an enterprise-ready hybrid application container.
Prerequisites
- Java JDK 6.
- Apache Ant 1.8 or later.
- Node.js.
- Android SDK version 21 or later.
- Note: For best results, install all previous versions of the Android SDK as well as your target version.
- Eclipse version 3.6 or later.
- Android Development Tools plugin for Eclipse version 21 or later.
- An Android Virtual Device (AVD) that targets Platform 2.2 or above (we recommend 4.0 or above).
- Sign up for a free Salesforce Platform Developer Edition (DE), if you don’t already have one. This will act as your own personal test environment. You should not use this tutorial with a trial, sandbox, or production org.
Create a Connected App in Salesforce
- Log into your Developer Edition.
- Open the Setup menu by clicking [Your Name] > Setup.
- Create a new Connected App by going to App Setup > Create > Apps.
- Click the ‘New’ button in the Connected Apps list.
- Fill out all required fields and click ‘Save’:
- Connected App: MySampleApp
- Developer Name: MySampleApp
- Contact Email: Your email
- Callback URL: sfdc://success.
- Selected all available OAuth scopes
- From the detail page of the connect app you just created, copy the Consumer Key and Callback URL as you’ll need these later.
-
Gourav
MemberJune 20, 2016 at 2:33 pm in reply to: How do we export dashboard into a PDF report in Salesforce?That´s a challenge. Here is how you should export dashboard into PDF report step by step:
- Create a new VisualForce Page
- Go to you dashboard and right click in the dashboard panel and select "Copy Image URL".
- Insert the copied url inside <img></img> tags on yous VisualForce Page
- Format the page code with lines, tables, text or anything else you want to
- Go to SETUP > CREATE > TAB. Create a new Visual Force Tab.
- Choose your new Visual Force page
- Since you can render the APEX PAGE as PDF, you will have the final result as follows.
-
Gourav
MemberJune 20, 2016 at 2:24 pm in reply to: Is there any way to Navigate back in Salesforce1, if I opened pdf in SalesForce1?Hi Piyush,
Add sforce.one.back(true); in your code it will help to redirect back to parent page after any function. Hope this will help you.
-
Gourav
MemberJune 20, 2016 at 2:04 pm in reply to: How can I get Bootstrap Table to correctly display records in Mobile? Works fine on Desktop and iPad for Salesforce.Hi Danna,
Try to use $A.util.addClass() to dynamically set css for different devices. it is salesforce util class to set css. Hope this will work for you . For more information please follow this link. https://developer.salesforce.com/docs/atlas.en-us.lightning.meta/lightning/js_cb_styles.htm
-
Gourav
MemberJune 20, 2016 at 1:58 pm in reply to: Bug: Redirect on to custom VisualForce page on click of record (Condition Based). How to fix this?This type of error indicates that an error has occurred that hasn't been trapped by the platform - a low-level java exception for example. All you can really do in terms of resolution is raise this with support and wait for a response.
If you need to move quicker than than, start removing functionality from the component to isolate where the problem occurs and see if there are any other mechanisms you can use.
-
Gourav
MemberJune 20, 2016 at 1:53 pm in reply to: How to select all checkboxes of wrapper list on vf page using jquery?Try to use this script in you VF page to select all check-box.
<script type="text/javascript">
function selectAllCheckboxes(obj,receivedInputID){
var inputCheckBox = document.getElementsByTagName("input");
for(var i=0; i<inputCheckBox.length; i++){
if(inputCheckBox[i].id.indexOf(receivedInputID)!=-1){
inputCheckBox[i].checked = obj.checked;
}
}
}
</script> -
The most scenario for this kind of error is when we forget to add __c after any custom field or if any customField __c (space) between them. Check your code again if this may be not the scenario.
-
You cannot query fields that are not existent in your org. First you'd have to create it either manually or using the api. Anyway in your test class you also need to create the records you want to test the query on. So you'd have something like:
List<Account> acclist = new List<Account>();
for(Integer i=0 ;i<200;i++){
Account a = new Account(Name='test'+i, customfield__c='abcd');
acclist.add(a);
}insert acclist;
List<Account> accList = Database.query('select Name,customfield__C
from Account');
System.debug(accList); -
Gourav
MemberJune 13, 2016 at 2:44 pm in reply to: Apex Page with analytics:reportChart in a Managed PackageHi
Try this link hope this will help you. It contains full description how to use dynamic reference for analytics:reportChart
OR
try this. it will not use reportId as filter try filtering on the Account Name and Site fields
<analytics:reportChart reportId="INSERT_REPORT_ID_HERE"
hideonerror="true"
filter="{column:'Account.Name', operator:'equals', value:'{!Visit_Preparation__c.Account__r.Name}'},{column:'Account.Site', operator:'equals', value:'{!Visit_Preparation__c.Account__r.Site}'}"
size="small"></analytics:reportChart> -
Gourav
MemberJune 13, 2016 at 2:24 pm in reply to: No more lookup filters in Managed Package context?I checked around and can't find confirmation of what happens to lookup filters in managed packages after passing the security review. But here's what I do know: Once your managed package passes the security review and you have it listed as public, you will be granted your own resource space, with the only exception (that I know of) being tabs. This changes if you have an Aloha App and then even tabs are not counted against the org you install in.
For example, in a privately listed, managed package that hasn't passed the security review yet, any triggers contained within will share the available resources with other triggers in the org (200 SOQL calls, 100 DML statements, 200K script statements, etc.) Once this managed package passes the review, the package gets its own set of 100 DML statements, 200 SOQL statements, 200K script statements, etc.
Although it's difficult to find specific documentation regarding these limit changes after completing the security review, I've been through the review process numerous times and experienced these changes firsthand - along with having confirmation of these changes from premier support. Although again, I haven't specifically checked for lookup filters in this regard.
So I would lean towards you getting your own set of lookup filter limits within your managed package - once certified. But I'm not 100% certain.
-
Gourav
MemberJune 13, 2016 at 2:10 pm in reply to: Create a User without asking them to set their password?Can you please explain the process of user creation? Are you trying to do Single Sign On with third party external system?
-
Try some time later again. Once this happen with me after few minutes I try again and it worked fine. If still issue persisted you can contact with salesforce and raise a case for this.
-
Gourav
MemberJune 13, 2016 at 2:07 pm in reply to: Can we update profile picture of user on onCreateUser()?Crate a method to updateAvatar(...) in this method you can use "ConnectApi.ChatterUsers.setPhoto method..." to update chatter avatar by twitter/fb avatar.
Hope this will help you!
-
The name must be unique for the current connected apps in your organization. You can reuse the name of a deleted connected app if the connected app was created using the Spring ’14 release or later. You cannot reuse the name of a deleted connected app if the connected app was created using an earlier release.
-
Gourav
MemberJune 10, 2016 at 1:34 pm in reply to: Email Alerts Not Log In Email Related list of CaseThis is currently missing functionality from salesforce. there is currently an idea on the success.salesforce.com site for this - Salesforce Success
The work around that we use here is to make sure you CC in your email to case email to get a copy into salesforce.
-
Gourav
MemberJune 10, 2016 at 1:32 pm in reply to: Best practice for new user notification when using Federated SSOBest practice is to
Turn on My Domain This creates a user-friendly URL which is the same for all users in the org: https://[mydomain].my.salesforce.com One of the advantages of MyDomain is that you can turn off non-SSO logins which lead to
Turn off 'native' SFDC login (aka Login Page authentication service) under Login Page Settings in My Domain. When your users go to https://[mydomain].my.salesforce.com , they'll be redirected to your SAML identity provider.
Note that anyone can still login with their SFDC username/password from https://login.salesforce.com but they no longer see the login page by default. Turning off login.salesforce.com is also possible via MyDomain.As far as how to notify the user, workflow-triggered email is a good way to go. The User object is usually not a good trigger point. To be productive in SF you typically need more than just a user account but perhaps your org is simple enough so you can trigger from User.
-
From trigger, you can not show the warning message. If you want to show the warning on the detail page of the record , just create a VF page and add it on the top of the detail page layout as inline page. Whenever your condition meets, that page will display the warning message
-
Gourav
MemberJune 10, 2016 at 1:23 pm in reply to: Navigating to URL from Visualforce page in Salesforce1Try to use Salesforce navigatetourl - sforce.one.navigateToURL
'javascript:sforce.one.navigateToURL(\''+ apexstr + warehouse.Id + '\')';
Hope this helps.