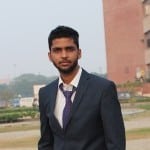
Vikas Kumar
IndividualForum Replies Created
-
Hi kumar ,
Go through following link
http://blog.jeffdouglas.com/2011/08/12/roll-your-own-salesforce-lookup-popup-window/
http://bobbuzzard.blogspot.in/2010/09/visualforce-lookup.html
Hope it may helps
Thanks
-
Vikas Kumar
MemberJanuary 16, 2017 at 7:10 am in reply to: Getting the current visualforce page name in SalesforceHi kumar,
Go through following link
-
Vikas Kumar
MemberJanuary 16, 2017 at 7:08 am in reply to: In Salesforce, how can we execute query in javascript function?Hi Sushant,
SOQL query in javascript example
You can use SOQL in java-script on your VF pages or any kind of java-script that you write, like we can get it executed on click of a button or link present on your detail page of a record. Below is the simple example and you can use it and modify it accordingly :{!REQUIRESCRIPT(“/soap/ajax/24.0/connection.js”)} {!REQUIRESCRIPT(“/soap/ajax/24.0/apex.js”)} try{ var query = “SELECT Id,Name from Account LIMIT 2″; var records = sforce.connection.query(query); var records1 = records.getArray(‘records’); alert(records); var accountNames = ”; for(var i=0;i<records1.length;i++){ accountNames = accountNames + records1[i].Name + ‘,’; } alert(accountNames); if(records1.length == 1){ //window.location.href = ‘http://www.google.com’; } else { alert(‘There is no Account’); } } catch(e){ alert(‘An Error has Occured. Error:’ +e); }
You need to use .js files that are in first two lines in order to use the api of salesforce to connect and fetch the records using SOQL. In the example you will see result of SOQL in alert statement. The result that is returned contains a ‘records’ named array component that can be used to iterate over and go through all the records and use it in the same manner as we do in usual apex program. For ex account.id, account.Name etc.
You can also use merge fields to create dynamic queries.
Similarly you can use javascript to create, update or delete the salesforce object’s records using API. Below is the sample code you can use to create a new account record in your org.
try{ var accounts = []; var account = new sforce.SObject("Account"); account.Name = "my new account Test"; accounts.push(account); var results = sforce.connection.create(accounts); if (results[0].getBoolean("success")) { alert("new account created with id " + results[0].id); } else { alert("failed to create account " + results[0]); } } catch(e){ alert('An Error has Occured. Error:' +e); }
-
Vikas Kumar
MemberJanuary 16, 2017 at 7:04 am in reply to: which one is better calling remote action or controller function on Salesforce Visualforce page?Hi sushant,
ACTION FUNCTION:
ActionFunction is used to execute a method in your Apex Class from within your Visualforce Page asynchronously via AJAX requests. What does asynchronous AJAX requests mean ? This means that Visualforce Pages(otherwise HTML pages when rendered at the Client Side via the Browser) can send data to, and retrieve data from, a server asynchronously (in the background) without interfering with the display and behavior of the existing page. So when we execute an Apex Method via the ActionFunction, the page is not disturbed and the request to the servers(Apex Code compiles and runs on the Salesforce servers while the Visualforce pages which are nothing but HTML pages are rendered by browser at the Client Side) are sent and received in the background. The other way of doing such AJAX requesst to Apex Methods include the Visualforce Remoting. The only difference between the same is that when using the Visualforce Remoting you will have to write some extra lines of JavaScript which is not needed in case of the ActionFunction.
Now, a very simple and a common example of ActionFunction is mimicking the Field Dependency feature on Visualforce Pages. Say for example you had two Picklists – Select the Object and Select the Field. When a user selects an Object, the next Picklist automatically gets populated with the Fields that belong to the object.
Well, I will not be using this one since that might get a bit complicated with couple of Describe calls but let us consider an another one. For example say you had two Picklists like this- Select the Alphabet(from which you can select from choices like –A, B, C and D) and then a Select the Fruit(from which you can select the fruit of your choice). So when a User selects A, all the Fruits starting with A gets populated in the 2nd picklist and when the User selects B, all the Fruits starting with B gets populated and so on.
CONTROLLER
public class Controller{
public List<SelectOption> Alphabets {get; set;}
public List<SelectOption> Fruits {get; set;}public String SelectedAlphabet {get; set;}
/*A Constructor which will build the intial list of Alphabets*/
public Controller(){
Alphabets = new List<SelectOption>();
Fruits = new List<SelectOption>();/*This is to add the NONE option for our Picklists*/
SelectOption option = new SelectOption('--None--', '--None--');
Alphabets.add(option);
Fruits.add(option);option = new SelectOption('A', 'A');
Alphabets.add(option);option = new SelectOption('B', 'B');
Alphabets.add(option);
}/*This Method that will actually build the Fruits list for us. The ActionFunction will be calling this function as and when a User changes an Alphabet from the 1st List.*/
public void createFruitList(){
/*Always clear the List when begin so that previous values will be removed.*/
Fruits.clear();Fruits.add(new SelectOption('--None--', 'None'));
if(SelectedAlphabet == 'A'){
Fruits.add(new SelectOption('Apple','Apple'));
Fruits.add(new SelectOption('Apricot','Apricot'));
}
else if(SelectedAlphabet == 'B'){
Fruits.add(new SelectOption('Banana','Banana'));
Fruits.add(new SelectOption('Blackberry','Blackberry'));
}
}
}VISUALFORCE PAGE
<apex:page controller="Controller">
<apex:form>
<apex:actionFunction action="{!createFruitList}" name="generateFruits" reRender="selFruits" />
<br/>
Select the Alphabet:
<apex:selectList id="selAlphabets" value="{!SelectedAlphabet}" size="1" onchange="generateFruits()">
<apex:selectOptions value="{!Alphabets}">
</apex:selectOptions>
</apex:selectList>
<br/>
Select the Fruit:
<apex:selectList id="selFruits" size="1">
<apex:selectOptions value="{!Fruits}">
</apex:selectOptions>
</apex:selectList>
</apex:form>
</apex:page>REMOTE ACTION:
@RemoteAction in Visual force page
JavaScript remoting in Visualforce provides support for some methods in Apex controllers to be called via JavaScript.JavaScript remoting has three parts:
The remote method invocation you add to the Visualforce page, written in JavaScript.
The remote method definition in your Apex controller class. This method definition is written in Apex, but there are few differences from normal action methods.
The response handler callback function you add to or include in your Visualforce page, written in JavaScript.
To use JavaScript remoting in a Visualforce page, add the request as a JavaScript invocation with the following form:[namespace.]controller.method(
[parameters...,]
callbackFunction,
[configuration]);
namespace is the namespace of the controller class. This is required if your organization has a namespace defined, or if the class comes from an installed package.
controller is the name of your Apex controller.
method is the name of the Apex method you’re calling.
parameters is the comma-separated list of parameters that your method takes.
callbackFunction is the name of the JavaScript function that will handle the response from the controller. You can also declare an anonymous function inline. callbackFunction receives the status of the method call and the result as parameters.
configuration configures the handling of the remote call and response. Use this to specify whether or not to escape the Apex method’s response. The default value is {escape: true}.VISUALFORCE PAGE
<apex:page controller="sample">
<script type="text/javascript">
function getAccountJS()
{
var accountNameJS = document.getElementById('accName').value;
sample.getAccount( accountNameJS,
function(result, event)
{
if (event.status)
{
// demonstrates how to get ID for HTML and Visualforce tags
document.getElementById("{!$Component.theBlock.thePageBlockSection.theFirstItem.accId}").innerHTML = result.Id;
document.getElementById("{!$Component.theBlock.thePageBlockSection.theSecondItem.accNam}").innerHTML = result.Name;
}
else if (event.type === 'exception')
{
document.getElementById("errors-js").innerHTML = event.message;
} else
{
document.getElementById("errors-js").innerHTML = event.message;
}
}, {escape:true});
}
</script>
Account Name :<input id="accName" type="text" />
<button onclick="getAccountJS()">Get Account</button>
<div id="errors-js"> </div>
<apex:pageBlock id="theBlock">
<apex:pageBlockSection id="thePageBlockSection" columns="2">
<apex:pageBlockSectionItem id="theFirstItem">
<apex:outputText id="accId"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem id="theSecondItem" >
<apex:outputText id="accNam" />
</apex:pageBlockSectionItem>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:page>CONTROLLER
global class sample
{
public String accountName { get; set; }
public static Account account { get; set; }
public sample() { }@RemoteAction
global static Account getAccount(String accountName)
{
account = [select id, name, phone, type, numberofemployees from Account where name = :accountName ];
return account;
}
} -
Vikas Kumar
MemberJanuary 16, 2017 at 6:58 am in reply to: When can we use REST API ,SOAP API,BULK API and STREAMING API in Salesforce?Hi sushant,
REST API
REST API is a simple and powerful web service based on REST ful principles. It exposes all sorts of Salesforce functionality via REST resources and HTTP methods. For example, you can create, read, update, and delete (CRUD) records, search or query your data, retrieve object metadata, and access information about limits in your org. REST API supports both XML and JSON.Because REST API has a lightweight request and response framework and is easy to use, it’s great for writing mobile and web apps.
SOAP API
SOAP API is a robust and powerful web service based on the industry-standard protocol of the same name. It uses a Web Services Description Language (WSDL) file to rigorously define the parameters for accessing data through the API. SOAP API supports XML only. Most of the SOAP API functionality is also available through REST API. It just depends on which standard better meets your needs.Because SOAP API uses the WSDL file as a formal contract between the API and consumer, it’s great for writing server-to-server integrations.
Bulk API
Bulk API is a specialized RESTful API for loading and querying lots of data at once. By lots, we mean 50,000 records or more. Bulk API is asynchronous, meaning that you can submit a request and come back later for the results. This approach is the preferred one when dealing with large amounts of data.Bulk API is great for performing tasks that involve lots of records, such as loading data into your org for the first time.
Streaming API
Streaming API is a specialized API for setting up notifications that trigger when changes are made to your data. It uses a publish-subscribe, or pub/sub, model in which users can subscribe to channels that broadcast certain types of data changes.The pub/sub model reduces the number of API requests by eliminating the need for polling. Streaming API is great for writing apps that would otherwise need to frequently poll for changes.
-
Vikas Kumar
MemberJanuary 11, 2017 at 7:19 am in reply to: Getting Error of FIELD_INTEGRITY_EXCEPTION while Executing Batch Class in salesforceHi sushant,
Thanks For the Help it works now
-
Vikas Kumar
MemberJanuary 10, 2017 at 8:23 am in reply to: Filtering results with NOT LIKE in Salesforce?Hi kumar,
you can try this query
select status, subject, whatId from task where NOT subject LIKE 'Email:%'
-
Vikas Kumar
MemberJanuary 10, 2017 at 8:22 am in reply to: When to use 'before events' and when to use 'after events' in Salesforce Triggers?Hello sushant,
see below is the difference between before and after trigger.
BEFORE trigger
BEFORE triggers are usually used when validation needs to take place before accepting the change. They run before any change is made to the database.BEFORE trigger
AFTER triggers are usually used when information needs to be updated in a separate table due to a change.
They run after changes have been made to the database (not necessarily committed).Generally we go for Before event if there is something needs to be validated before the actual data is commited to database.
For example :
Let suppose you want to Insert new Account, but before the date is commited to database you want to validate if the Billing city is not null, if Null you will throw error and or else you will Insert record.
And in case of After, once the record is inserted/ Updated or Deleted, based on some condition you want to do some process.
For example:
Once the record is Inserted you want to send mail to update some filed on object "XYZ". -
Vikas Kumar
MemberJanuary 10, 2017 at 8:18 am in reply to: Is there any other formatting language available in salesforce, other than JSON and XML in API ?Hi Sushant,
Yes you can get response also in Html,Audio,Text its depends on your api what type of response you wanted to send
hope it may help you
thanks
-
Vikas Kumar
MemberJanuary 10, 2017 at 8:12 am in reply to: Can not create class from enterprise WSDL file in SalesforceHi kumar,
you need to manually trim your wsdl or get a class from outsource software and save it in your org
-
Vikas Kumar
MemberJanuary 10, 2017 at 8:09 am in reply to: How to edit global picklists in Salesforce?Hi kumar,
we can easily edit global picklist for this
From Setup, enter Picklists in the Quick Find box, then select Picklist Value Sets.
Next to Global Value Sets, click edit click saveHope it may helps
-
Vikas Kumar
MemberJanuary 9, 2017 at 12:43 pm in reply to: Difference between OAuth 1 and OAuth 2 in Salesforce?Hi kumar,
I find the following diagrams very useful. They illustrate the difference in communication between parties with OAuth2 and OAuth1.
OAuth2
OAuth1
-
This reply was modified 7 years, 3 months ago by
Vikas Kumar.
-
This reply was modified 7 years, 3 months ago by
-
Vikas Kumar
MemberJanuary 9, 2017 at 12:39 pm in reply to: What is the difference between http methods PUT and POST in Salesforce?Hi sushant,
HTTP PUT:
PUT puts a file or resource at a specific URI, and exactly at that URI. If there's already a file or resource at that URI, PUT replaces that file or resource. If there is no file or resource there, PUT creates one. PUT is idempotent, but paradoxically PUT responses are not cacheable.
HTTP POST:
POST sends data to a specific URI and expects the resource at that URI to handle the request. The web server at this point can determine what to do with the data in the context of the specified resource. The POST method is not idempotent, however POST responses are cacheable so long as the server sets the appropriate Cache-Control and Expires headers.
The official HTTP RFC specifies POST to be:
Annotation of existing resources;
Posting a message to a bulletin board, newsgroup, mailing list, or similar group of articles;
Providing a block of data, such as the result of submitting a form, to a data-handling process;
Extending a database through an append operation. -
Vikas Kumar
MemberJanuary 9, 2017 at 12:30 pm in reply to: How is Access Token used with REST API in Salesforce ?Hi sushant,
Access token is used for authorization purpose.
Once authenticated, every request must pass in the access_token value in the header. It cannot be passed as a request parameter.
go through https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_oauth.htm for detail option
Hope it may help
-
Hi kumar,
you can use json.deserialize() for converting json content into standard object or customobject
deserialize(jsonString, apexType)
Deserializes the specified JSON string into an Apex object of the specified type.
Signature
public static Object deserialize(String jsonString, System.Type apexType)
Parameters
jsonString
Type: String
The JSON content to deserialize.
apexType
Type: System.Type
The Apex type of the object that this method creates after deserializing the JSON content.
Return Value
Type: Object
Usage
If the JSON content contains attributes not present in the System.Type argument, such as a missing field or object, deserialization fails in some circumstances. When deserializing JSON content into a custom object or an sObject using Salesforce API version 34.0 or earlier, this method throws a runtime exception when passed extraneous attributes. When deserializing JSON content into an Apex class in any API version, or into an object in API version 35.0 or later, no exception is thrown. When no exception is thrown, this method ignores extraneous attributes and parses the rest of the JSON content. -
Vikas Kumar
MemberJanuary 9, 2017 at 12:15 pm in reply to: How can we make batch class iterable in Salesforce?Hi sushant,
We can make batch iterable by calling batch again inside finish method for more detail go through below code
global class iterablebatch implements Database.Batchable<sobject>{
global String Query;
global List<id>allObjIds ;
global string idInitials;
global iterablebatch(List<id>allObjectIds,String var ){
allObjIds=allObjectIds;
idInitials =var;
}
global Database.QueryLocator Start(Database.BatchableContext BC){
if (idInitials=='001')
query='SELECT Id,Name FROM Account WHERE Id in:allObjIds';
if(idInitials=='003')
query='SELECT Id,Name FROM Contact WHERE Id in:allObjIds';
if(idInitials=='006')
query='SELECT Id,Name FROM Opportunity WHERE Id in:allObjIds';
return Database.getQueryLocator(query);
}
global void execute (Database.BatchableContext BC,List<Sobject>scope){
Schema.SObjectType sObjectType =scope.getSObjectType();
String listType = 'List<' + sObjectType + '>';
List<SObject> castRecords = (List<SObject>)Type.forName(listType).newInstance();
castRecords.addAll(scope);
if (idInitials=='001'){for(sobject obj:castRecords){
Account acc= (account) obj;acc.Name='Algoworks1';
}}
if (idInitials=='003'){for(sobject obj:castRecords){
contact con=(contact)obj;
con.LastName='Algoworks1';}
}
if (idInitials=='006'){for(sobject obj:castRecords){
opportunity opp=(opportunity)obj;
opp.Name='Algoworks1'; }
}
update scope;
}
global void finish (Database.BatchableContext BC){
iterablebatch objc = new iterablebatch(allObjIds,'003');
iterablebatch objo = new iterablebatch(allObjIds,'006');}
}Hope it may helps
-
Vikas Kumar
MemberJanuary 9, 2017 at 12:10 pm in reply to: What is aura in Salesforce lightning experience ?Hi sushant,
Aura is a framework for developing apps for mobile and desktop devices. In existence since 2013, Aura enables developers to build apps that are independent of data that resides in Salesforce, so development can be less disruptive to day-to-day users. Aura also enables efficient development because applications don't need to be optimized per device. -
Vikas Kumar
MemberJanuary 9, 2017 at 12:05 pm in reply to: What is journey builder in salesforce's marketing cloud? What are the uses of it?Hi sushant ,
Salesforce Journey Builder is a feature of the company's Marketing Cloud that manages the customer life cycle: the progression of steps a customer goes through when considering, purchasing, using and maintaining loyalty to a brand.
Salesforce conceptualizes the progression of interactions as a journey through customer relationship stages including reach, acquisition, conversion, retention and loyalty. Journey Builder is designed to allow marketers to customize interactions through those stages based on customer needs, desires and preferences, their demographics and real-time input from their behavior.
Journey Builder uses event-driven triggers to respond to customers or prospective customers appropriately. Events can include anything relevant that the customer does: Downloading an app or a product fact sheet, abandoning a shopping cart, joining a loyalty program or triggering a beacon in a physical location. The software accesses information about the customer to guide the responses; those events and the responses to them are all part of the customer journey, which becomes longer and more detailed with every interaction. Triggers can automatically update the customer's contact data and establish "decision splits" to enable the appropriate action to take in real-time, based on the customer's behavior.
-
Vikas Kumar
MemberJanuary 9, 2017 at 11:34 am in reply to: Formula to tell day for a given date in SalesforceHi kumar,
use this
CASE( MOD( Date__c - DATE(1900, 1, 7), 7), 0, "Sunday", 1, "Monday", 2, "Tuesday", 3,
"Wednesday", 4, "Thursday", 5, "Friday", 6, "Saturday","Error")hope it may helps
-
Vikas Kumar
MemberJanuary 9, 2017 at 11:32 am in reply to: Difference between ISBLANK and ISNULL in SalesforceHi kumar,
ISBLANK has the same functionality as ISNULL, but also supports text fields.
Text fields are never null, so using ISNULL() with a text field always returns false. For example, the formula field IF(ISNULL(new__c) 1, 0) is always zero regardless of the value in the New field. For text fields, use the ISBLANK function instead.
-
Hi kumar ,
A webhook (also called a web callback or HTTP push API) is a way for an app to provide other applications with real-time information. A webhook delivers data to other applications as it happens, meaning you get data immediately.
The first step in consuming a webhook is giving the webhook provider a URL to deliver requests to. This is most often done through a backend panel or an API. This means that you also need to set up a URL in your app that’s accessible from the public web.
The majority of webhooks will POST data to you in one of two ways: as JSON or XML to be interpreted, or as a form data (application/x-www-form-urlencoded or multipart/form-data). Your provider will tell you how they deliver it (or even give you a choice in the matter). Both of these are fairly easy to interpret, and most web frameworks will do the work for you. If they don’t, you may need to call on a function or two.
for more info go through https://www.jamesward.com/2014/06/30/create-webhooks-on-salesforce-com
-
Vikas Kumar
MemberJanuary 9, 2017 at 11:22 am in reply to: In Salesforce, how to make an unmanaged package to managed package in DEV org?Hi sushant,
Go through below link for the procedure
Thanks
-
Vikas Kumar
MemberJanuary 9, 2017 at 11:18 am in reply to: How can we map an object field to another field of different target org in Talend?Hi Sushant,
we can map an object field to another field of different target org in Talend by using t map component.
tMap component will map the input schema to the output schema.
-
Vikas Kumar
MemberJanuary 9, 2017 at 11:14 am in reply to: Searching contents of Attachments in salesforceHi kumar,
If you upload a document as a "file" (this is store as Chatter>Files>Document Name) the content becomes searchable. However this file cannot be linked to a contact record.
you can implement this by the help of triggers for more check https://developer.salesforce.com/forums/ForumsMain?id=906F0000000AaMqIAK
Hape it may helps
-
Hi sushant,
The tMap component is part of the Processing family of components. tMap is one of the core components and is primarily used for mapping input data to output data, that is, mapping one Schema to another.As well as performing mapping functions, tMap may also be used to Join multiple inputs, and to write multiple outputs. Additionally, you can Filter data within the tMap component.